nodejs使用http-proxy库实现多个域名代理和同时代理websocket的例子,代理包含https和http两种协议
2023/03/23
nodejs使用http-proxy库实现多个域名代理和同时代理websocket的例子,代理包含https和http两种协议
以下是使用http-proxy库实现多个域名代理和同时代理websocket的例子,代理包含https和http两种协议的代码示例:
javascript const http = require('http'); const https = require('https'); const httpProxy = require('http-proxy'); // 创建代理服务器 const proxy = httpProxy.createProxyServer({ ws: true, // 同时代理websocket }); // 监听代理错误事件 proxy.on('error', (err, req, res) => { console.error(err); res.writeHead(500, { 'Content-Type': 'text/plain' }); res.end('代理服务器出错'); }); // 创建http服务器 const server = http.createServer((req, res) => { // 根据请求的域名进行代理配置 switch (req.headers.host) { case 'www.example.com': proxy.web(req, res, { target: 'http://localhost:3000' }); break; case 'api.example.com': proxy.web(req, res, { target: 'http://localhost:4000' }); break; default: res.writeHead(404, { 'Content-Type': 'text/plain' }); res.end('未找到对应的代理服务器'); } }); // 监听http服务器错误事件 server.on('error', (err) => { console.error(err); }); // 创建https服务器 const options = { key: fs.readFileSync('ssl/private.key'), cert: fs.readFileSync('ssl/certificate.crt') }; const secureServer = https.createServer(options, (req, res) => { // 根据请求的域名进行代理配置 switch (req.headers.host) { case 'www.example.com': proxy.web(req, res, { target: 'https://localhost:3001', secure: false }); break; case 'api.example.com': proxy.web(req, res, { target: 'https://localhost:4001', secure: false }); break; default: res.writeHead(404, { 'Content-Type': 'text/plain' }); res.end('未找到对应的代理服务器'); } }); // 监听https服务器错误事件 secureServer.on('error', (err) => { console.error(err); }); // 同时监听http和https服务器 server.listen(80); secureServer.listen(443);
以上代码中,使用http-proxy库创建了一个代理服务器,并监听代理错误事件。然后创建了一个http服务器和一个https服务器,根据请求的域名进行代理配置,将请求转发到不同的目标服务器。同时代理websocket需要设置`ws`为true。
注意:在使用https代理时,需要传入证书和私钥,且需要将`secure`参数设置为false,否则会出现证书验证错误。
上一篇:
nodejs 操作数据库的库
下一篇:
electron mac打包注意问题
静水缘首页
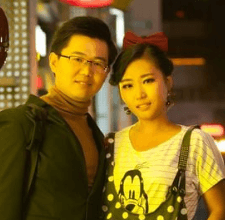
文章分类
最新文章
- nodejs私钥加密公钥解密的一个例子
- uniapp和微信小程序判断程序运行在开发或者测试或者线上版本的方法分别是什么
- electron使用electron-builder打包后模块包含exe文件执行失败
- Compile is disallowed on the main thread, if the buffer size is larger than 4KB
- better-sqlite3简介及常用操作
- nodejs 操作数据库的库
- nodejs使用http-proxy库实现多个域名代理和同时代理websocket的例子,代理包含https和http两种协议
- iis配置反向代理
- javascript伪多线程代码
- ip所在地址段判断