c#图片完美裁剪,新闻展示
2018/11/14
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Text; using System.IO; using System.Drawing; using System.Drawing.Drawing2D; using System.Drawing.Imaging; using System.Text.RegularExpressions; public partial class Default2 : System.Web.UI.Page { /// <summary> /// 图片处理 /// http://www.lpsjj.cn/ /// /// 刘丕水 2018-11-14 创建 /// </summary> public class Image { /// <summary> /// 图片展示完美裁剪 /// </summary> /// <remarks>刘丕水 2018-11-14</remarks> /// <param name="fromFile">原图Stream对象</param> /// <param name="savePath">缩略图存放地址</param> /// <param name="targetWidth">指定的最大宽度</param> /// <param name="targetHeight">指定的最大高度</param> /// <param name="watermarkText">水印文字(为""表示不使用水印)</param> /// <param name="watermarkImage">水印图片路径(为""表示不使用水印)</param> public static byte[] cutPerfect(System.IO.Stream fromFile, System.Double targetWidth, System.Double targetHeight, string watermarkText, string watermarkImage) { //原始图片(获取原始图片创建对象,并使用流中嵌入的颜色管理信息) System.Drawing.Image initImage = System.Drawing.Image.FromStream(fromFile, true); MemoryStream ms = new MemoryStream(); //缩略图宽、高计算 double newWidth = (int)targetWidth; double newHeight = (int)targetHeight; int startX = 0; int startY = 0; if ((int)targetWidth*initImage.Height/((int)targetHeight*initImage.Width)<1) { newWidth = (int)targetHeight * initImage.Width / initImage.Height; startX = (int)(((int)targetWidth - newWidth) / 2); } else { newHeight = (int)targetWidth*initImage.Height/initImage.Width; startY = (int)(((int)targetHeight - newHeight) / 2); } //新建一个bmp图片 System.Drawing.Image newImage = new System.Drawing.Bitmap((int)targetWidth, (int)targetHeight); //新建一个画板 System.Drawing.Graphics newG = System.Drawing.Graphics.FromImage(newImage); //设置质量 newG.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.HighQualityBicubic; newG.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.HighQuality; //置背景色 newG.Clear(Color.White); //画图 newG.DrawImage(initImage, new System.Drawing.Rectangle(startX, startY, (int)newWidth, (int)newHeight), new System.Drawing.Rectangle(0, 0, initImage.Width, initImage.Height), System.Drawing.GraphicsUnit.Pixel); //文字水印 if (watermarkText != "") { using (System.Drawing.Graphics gWater = System.Drawing.Graphics.FromImage(newImage)) { int fontSizeCurr = 15; System.Drawing.Font fontWater = new Font("宋体", fontSizeCurr,FontStyle.Italic); System.Drawing.Brush brushWater = new SolidBrush(Color.FromArgb(150,0,0,0)); gWater.DrawString(watermarkText, fontWater, brushWater, (int)targetWidth-60-watermarkText.Length* fontSizeCurr, (int)targetHeight - 30- fontSizeCurr); gWater.Dispose(); } } //透明图片水印 if (watermarkImage != "") { if (File.Exists(watermarkImage)) { //获取水印图片 using (System.Drawing.Image wrImage = System.Drawing.Image.FromFile(watermarkImage)) { //水印绘制条件:原始图片宽高均大于或等于水印图片 if (newImage.Width >= wrImage.Width && newImage.Height >= wrImage.Height) { Graphics gWater = Graphics.FromImage(newImage); //透明属性 ImageAttributes imgAttributes = new ImageAttributes(); ColorMap colorMap = new ColorMap(); colorMap.OldColor = Color.FromArgb(255, 0, 255, 0); colorMap.NewColor = Color.FromArgb(0, 0, 0, 0); ColorMap[] remapTable = { colorMap }; imgAttributes.SetRemapTable(remapTable, ColorAdjustType.Bitmap); float[][] colorMatrixElements = { new float[] {1.0f, 0.0f, 0.0f, 0.0f, 0.0f}, new float[] {0.0f, 1.0f, 0.0f, 0.0f, 0.0f}, new float[] {0.0f, 0.0f, 1.0f, 0.0f, 0.0f}, new float[] {0.0f, 0.0f, 0.0f, 0.5f, 0.0f},//透明度:0.5 new float[] {0.0f, 0.0f, 0.0f, 0.0f, 1.0f} }; ColorMatrix wmColorMatrix = new ColorMatrix(colorMatrixElements); imgAttributes.SetColorMatrix(wmColorMatrix, ColorMatrixFlag.Default, ColorAdjustType.Bitmap); gWater.DrawImage(wrImage, new Rectangle(newImage.Width - wrImage.Width, newImage.Height - wrImage.Height, wrImage.Width, wrImage.Height), 0, 0, wrImage.Width, wrImage.Height, GraphicsUnit.Pixel, imgAttributes); gWater.Dispose(); } wrImage.Dispose(); } } } //保存缩略图到流 newImage.Save(ms,System.Drawing.Imaging.ImageFormat.Jpeg); //释放资源 newG.Dispose(); newImage.Dispose(); initImage.Dispose(); //ms流数据转换为Buffer数据; long fileSize = ms.Length; byte[] fileBuffer = new byte[fileSize]; ms.Position = 0; //流在输出之前一定要先定位到0.否则.读出的数据全都是0 ms.Read(fileBuffer, 0, (int)fileSize); //如果不写ms.Close()语句,用户在下载过程中选择取消,将不能再次下载 ms.Close(); return fileBuffer; } #region 其它 /// <summary> /// 判断文件类型是否为WEB格式图片 /// (注:JPG,GIF,BMP,PNG) /// </summary> /// <param name="contentType">HttpPostedFile.ContentType</param> /// <returns></returns> public static bool IsWebImage(string contentType) { if (contentType == "image/pjpeg" || contentType == "image/jpeg" || contentType == "image/gif" || contentType == "image/bmp" || contentType == "image/png") { return true; } else { return false; } } #endregion }//end class protected void Page_Load(object sender, EventArgs e) { Response.AddHeader("content-type", "image/png"); string imgPath = HttpContext.Current.Request["path"].ToString(); string serverPath = Server.MapPath(""); string imgPathPre = ""; string imgPathNext = ""; string imgPathType = ""; int imgSizeWidth=0; int imgSizeHeight=0; imgPathPre = imgPath.Substring(0,imgPath.LastIndexOf("_")); imgPathNext = imgPath.Substring(imgPath.LastIndexOf("_")+1); imgPathType = imgPath.Split('.')[1]; imgSizeWidth = int.Parse(imgPathNext.Split('.')[0].Split('x')[0]); imgSizeHeight = int.Parse(imgPathNext.Split('.')[0].Split('x')[1]); Stream imgStream = File.Open(serverPath + "/" + imgPathPre + "." + imgPathType, FileMode.Open); byte[] imgBtye = Image.cutPerfect(imgStream, imgSizeWidth, imgSizeHeight, "刘丕水", ""); imgStream.Close(); Response.BinaryWrite(imgBtye); Response.OutputStream.Flush(); Response.OutputStream.Close(); } }
下一篇:
多行文字截断(多余文字省略号显示)
静水缘首页
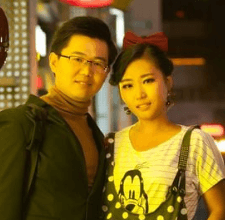
文章分类
最新文章
- nodejs私钥加密公钥解密的一个例子
- uniapp和微信小程序判断程序运行在开发或者测试或者线上版本的方法分别是什么
- electron使用electron-builder打包后模块包含exe文件执行失败
- Compile is disallowed on the main thread, if the buffer size is larger than 4KB
- better-sqlite3简介及常用操作
- nodejs 操作数据库的库
- nodejs使用http-proxy库实现多个域名代理和同时代理websocket的例子,代理包含https和http两种协议
- iis配置反向代理
- javascript伪多线程代码
- ip所在地址段判断